05:00
From R Markdown to Quarto
UC Berkeley
Duke University + Posit
Where does the name “Quarto” come from?
Metadata: YAML
Text: Markdown
Code: Executed via knitr
or jupyter
Weave it all together, and you have beautiful, powerful, and useful outputs!
Literate programming is writing out the program logic in a human language with included code snippets (separated by a primitive markup) and macros.
“Yet Another Markup Language” or “YAML Ain’t Markup Language” is used to provide document level metadata …
… in key-value pairs,
… that can nest,
… are fussy about indentation,
Indentation matters!
:
There are multiple ways of formatting valid YAML:
:
:
format: html
with additional options made with proper indentationTo avoid manually typing out all the options, every time when rendering via the CLI:
Lint, or a linter, is a static code analysis tool used to flag programming errors, bugs, stylistic errors and suspicious constructs.
RStudio + VSCode provide rich tab-completion - start a word and tab to complete, or Ctrl + space
to see all available options.
hello-penguins.qmd
in RStudio.Ctrl + space
to see the available YAML options.05:00
Many YAML fields are common across various outputs
But also each output type has its own set of valid YAML fields and options
Definitive list: quarto.org/docs/reference/formats/html
Markdown Syntax | Output |
---|---|
|
italics and bold |
|
superscript2 / subscript2 |
|
|
|
verbatim code |
Markdown Syntax | Output |
---|---|
|
Header 1 |
|
Header 2 |
|
Header 3 |
|
Header 4 |
|
Header 5 |
|
Header 6 |
There are several types of “links” or hyperlinks.
Markdown
Output
You can embed named hyperlinks, direct urls like https://quarto.org/, and links to other places in the document. The syntax is similar for embedding an inline image: .
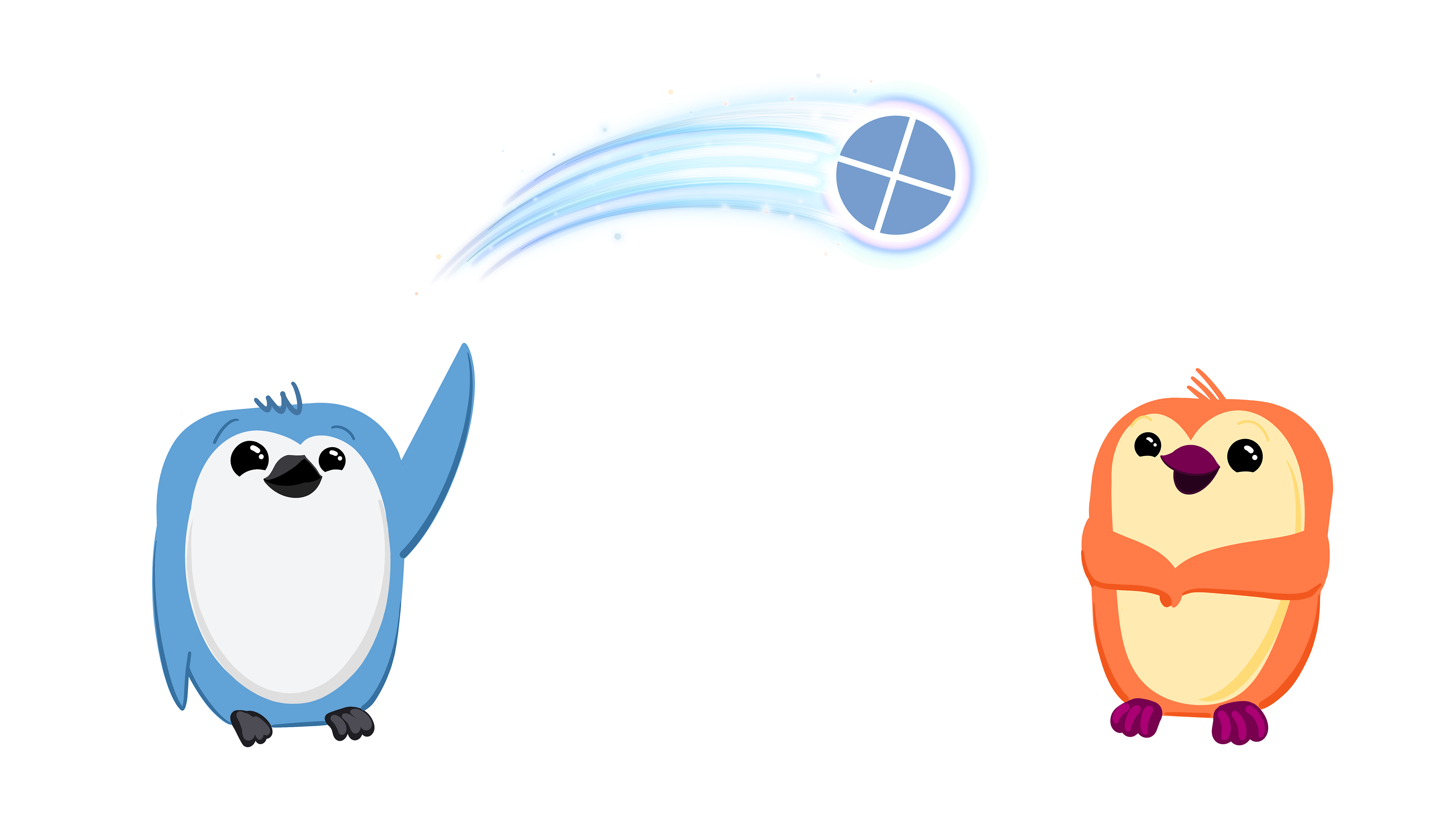
Penguins playing with a Quarto ball
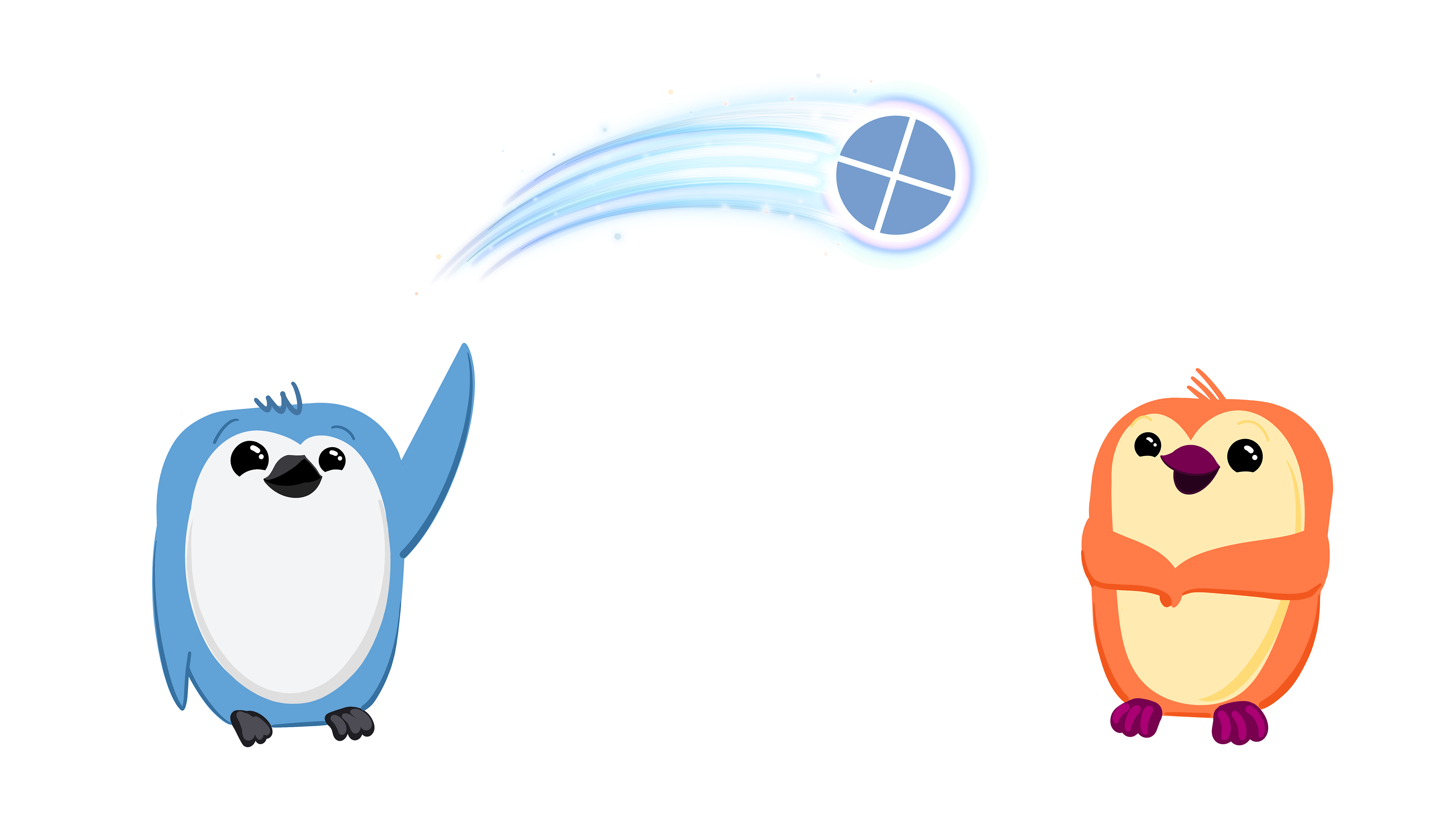{fig-align="left" width=250}
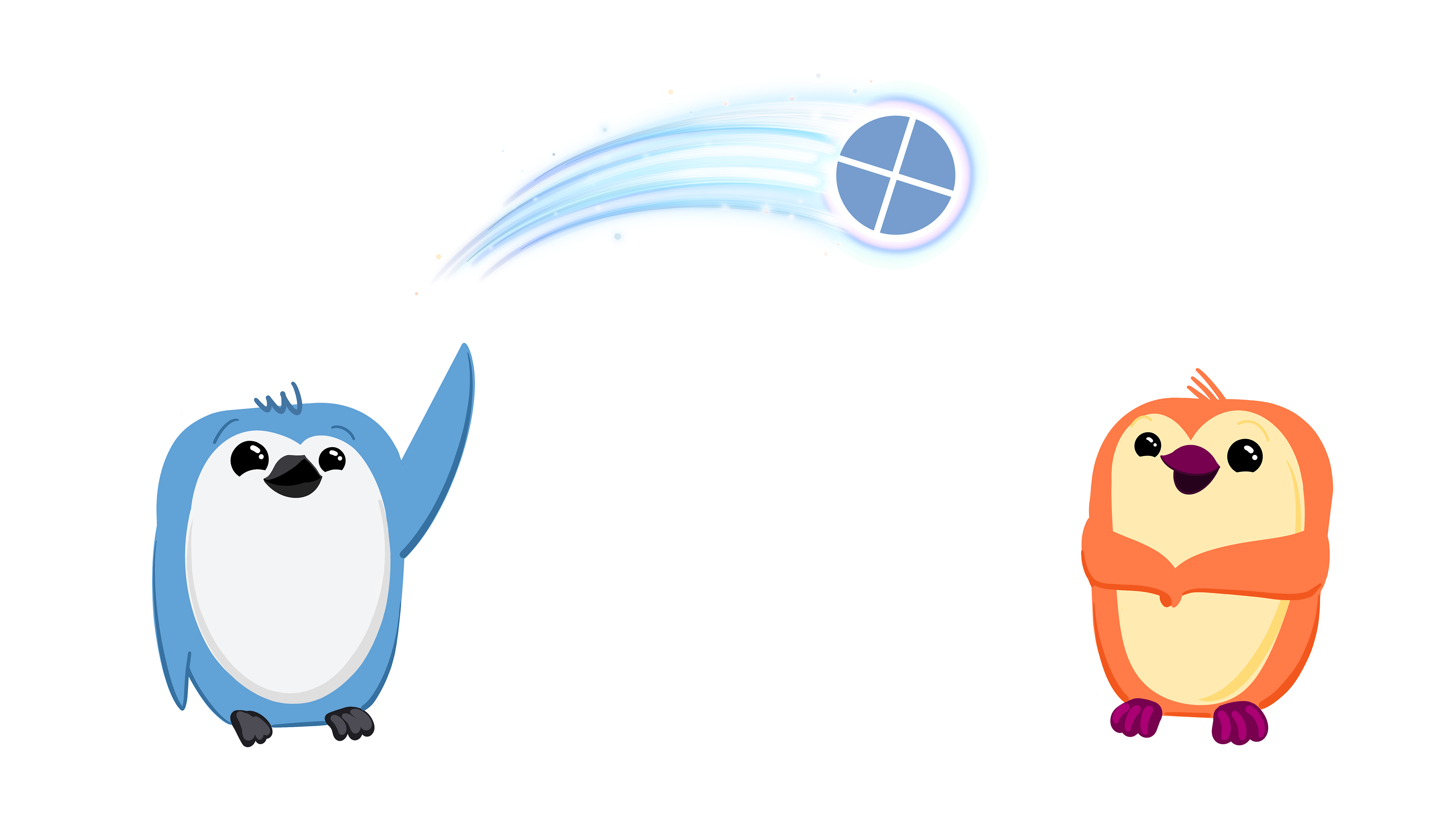{fig-align="right" width=250 fig-alt="Illustration of two penguins playing with a Quarto ball."}
Unordered list:
Output
Ordered list:
Markdown:
> Let us change our traditional attitude to the construction of programs: Instead of imagining that our main task is to instruct a computer what to do, let us concentrate rather on explaining to human beings what we want a computer to do.
> - Donald Knuth, Literate Programming
Output:
Let us change our traditional attitude to the construction of programs: Instead of imagining that our main task is to instruct a computer what to do, let us concentrate rather on explaining to human beings what we want a computer to do. - Donald Knuth, Literate Programming
markdown-syntax.qmd
in RStudio.05:00
Markdown:
| Right | Left | Default | Center |
|------:|:-----|---------|:------:|
| 12 | 12 | 12 | 12 |
| 123 | 123 | 123 | 123 |
| 1 | 1 | 1 | 1 |
Output:
Right | Left | Default | Center |
---|---|---|---|
12 | 12 | 12 | 12 |
123 | 123 | 123 | 123 |
1 | 1 | 1 | 1 |
Markdown:
+---------------+---------------+--------------------+
| Fruit | Price | Advantages |
+===============+===============+====================+
| Bananas | $1.34 | - built-in wrapper |
| | | - bright color |
+---------------+---------------+--------------------+
| Oranges | $2.10 | - cures scurvy |
| | | - tasty |
+---------------+---------------+--------------------+
: Sample grid table.
Output:
Fruit | Price | Advantages |
---|---|---|
Bananas | $1.34 |
|
Oranges | $2.10 |
|
+---------------+---------------+--------------------+
| Right | Left | Centered |
+==============:+:==============+:==================:+
| Bananas | $1.34 | built-in wrapper |
+---------------+---------------+--------------------+
+--------------:+:--------------+:------------------:+
| Right | Left | Centered |
+---------------+---------------+--------------------+
Note that grid tables are quite awkward to write with a plain text editor because unlike pipe tables, the column indicators must align.
The Visual Editor can assist in making these tables!
The knitr package can turn data frames into tables with knitr::kable()
:
species | island | bill_length_mm | bill_depth_mm | flipper_length_mm | body_mass_g | sex | year |
---|---|---|---|---|---|---|---|
Adelie | Torgersen | 39.1 | 18.7 | 181 | 3750 | male | 2007 |
Adelie | Torgersen | 39.5 | 17.4 | 186 | 3800 | female | 2007 |
Adelie | Torgersen | 40.3 | 18.0 | 195 | 3250 | female | 2007 |
Adelie | Torgersen | NA | NA | NA | NA | NA | 2007 |
Adelie | Torgersen | 36.7 | 19.3 | 193 | 3450 | female | 2007 |
Adelie | Torgersen | 39.3 | 20.6 | 190 | 3650 | male | 2007 |
If you want fancier tables, try the gt package and all that it offers!
species | island | bill_length_mm | bill_depth_mm | flipper_length_mm | body_mass_g | sex | year |
---|---|---|---|---|---|---|---|
Adelie | Torgersen | 39.1 | 18.7 | 181 | 3750 | male | 2007 |
Adelie | Torgersen | 39.5 | 17.4 | 186 | 3800 | female | 2007 |
Adelie | Torgersen | 40.3 | 18.0 | 195 | 3250 | female | 2007 |
Adelie | Torgersen | NA | NA | NA | NA | NA | 2007 |
Adelie | Torgersen | 36.7 | 19.3 | 193 | 3450 | female | 2007 |
Adelie | Torgersen | 39.3 | 20.6 | 190 | 3650 | male | 2007 |
Help readers to navigate your document with numbered references and hyperlinks to entities like figures and tables.
Cross referencing steps:
fig-
or tbl-
.@fig-...
or @tbl-...
.The presence of the caption (Blue penguin
) and label (#fig-blue-penguin
) make this figure referenceable:
Markdown:
The presence of the caption (A few penguins
) and label (#tbl-penguins
) make this table referenceable:
Markdown:
Output:
See Table 1 for data on a few penguins.
species | island | bill_length_mm | bill_depth_mm | flipper_length_mm | body_mass_g | sex | year |
---|---|---|---|---|---|---|---|
Adelie | Torgersen | 39.1 | 18.7 | 181 | 3750 | male | 2007 |
Adelie | Torgersen | 39.5 | 17.4 | 186 | 3800 | female | 2007 |
Adelie | Torgersen | 40.3 | 18.0 | 195 | 3250 | female | 2007 |
Adelie | Torgersen | NA | NA | NA | NA | NA | 2007 |
Adelie | Torgersen | 36.7 | 19.3 | 193 | 3450 | female | 2007 |
Adelie | Torgersen | 39.3 | 20.6 | 190 | 3650 | male | 2007 |
The presence of the caption (A few penguins
) and label (#tbl-penguins
) make this table referenceable:
Markdown:
Output:
See Table 2 for data on a few penguins.
Right | Left |
---|---|
12 | 12 |
123 | 123 |
tables-figures.qmd
.08:00
Fenced Div and Bracketed Span
Use case: highlight content for the reader in multiple formats.
Highlight content for the reader in multiple formats.
Highlight content for the reader in multiple formats.
What syntax is being used in echo=FALSE
?
How can this be generalized to other languages?
How can this be generalized to other languages?
How can this be generalized to other languages?
#|
{r, and=on, and=on, and=on, and=on, and=on, and=on}
<commentchar>|
.eval=FALSE
)Control how the code is executed with options.
Option | Description |
---|---|
eval |
Evaluate the code chunk (if false , just echos the code into the output). |
echo |
Include the source code in output |
output |
Include the results of executing the code in the output (true , false , or asis to indicate that the output is raw markdown and should not have any of Quarto’s standard enclosing markdown). |
warning |
Include warnings in the output. |
error |
Include errors in the output. |
include |
Catch all for preventing any output (code or results) from being included (e.g. include: false suppresses all output from the code block). |
Don’t forget to use cmd-space to see the available options!
Options can be moved into YAML under the execute
key to apply to all chunks. Exceptions to that option can be set cell-by-cell.
You can also pass options via YAML to knitr through the knitr
key1.
Options can be moved into YAML under the execute
key to apply to all chunks. Exceptions to that option can be set cell-by-cell.
You can also pass options via YAML to knitr through the knitr
key1.
You can use knitr to pass options that control your R session.
```{r}
#| fig-width: 5
#| fig-height: 3
#| fig-cap: "Size of penguins on three islands in the Palmer Archipelago."
#| fig-alt: "Scatterplot showing the bill sizes of penguins across three islands."
library(palmerpenguins)
library(ggplot2)
ggplot(penguins, aes(x = bill_length_mm,
y = bill_depth_mm,
col = island)) +
geom_point()
```
Save time/code by moving figure sizing defaults up to the YAML.
callout-boxes.qmd
and render the document.{ }
to learn what they do.
icon=true
or icon=false
.appearance="simple"
(can also try "minimal"
and "default"
).05:00
code-cells.qmd
and render the document.echo: false
to the code cell and re-render.#|
or consult the Quarto Reference.07:00